The Java programs are frequently asked in the interview whether you are fresher or Experienced.
Java programs are useful for logic building as well as cracking interviews.
If you are fresher in Java, we recommend you to read our Java programs, and implement them with your own logic, so that you can build logic on how to implement particular things.
They can be asked from control statements, array, string, oops, etc. Java basic programs like the Fibonacci series, prime numbers, factorial numbers, and palindrome numbers are frequently asked in interviews and exams.
Programs can be asked from:
- Arrays & Strings
- Loops & Conditional Statements
- Static and Non-Static methods
- Exception Handling & Error Handling
- Throw Exceptions
- Interface and Classes in Java
- Inheritance Concepts
- Collections
Java programs are frequently asked in interviews.
Simple Java Program to print Hello World
package com.asyncster.java.program.examples;
/*
Simple Java Program to print Hello World
*/
public class HelloWorld {
public static void main(String args[]) {
System.out.println("Hello World JavaCodeStuffs!!!");
}
}
Java Program to Print numbers from 1 to 10
package com.asyncster.java.program.examples;
/*
Java Program to Print number from 1 to 10
*/
public class PrintIntegers {
public static void main(String[] arguments) {
System.out.println("the integers between 1 to 10 are : ");
for (int count = 1; count <= 10; count++) {
System.out.println(count);
}
}
}
the integers between 1 to 10 are :
1
2
3
4
5
6
7
8
9
10
Java program to find the number is even or Odd
package com.asyncster.java.program.examples;
import java.util.Scanner;
/*Java program to the find number is even or Odd.
*/
public class FindEvenOrOdd {
public static void main(String[] args) {
int num;
System.out.println("Enter the number to find even or Odd : ");
Scanner scanner = new Scanner(System.in);
num = scanner.nextInt();
if (num % 2 == 0) {
System.out.println("The number " + num + " is a Even Number.");
} else {
System.out.println("The number " + num + " is a Odd Number.");
}
scanner.close();
}
}
Enter the number to find even or Odd :
223
The number 223 is a Odd Number.
Java Program to convert Fahrenheit to Celsius
package com.asyncster.java.program.examples;
/*Java Program to convert Fahrenheit to Celsius
*/
import java.util.*;
public class FahrenheitToCelsius {
public static void main(String[] args) {
float temperatue;
Scanner scanner = new Scanner(System.in);
System.out.println("Enter temperatue in Fahrenheit : ");
temperatue = scanner.nextInt();
temperatue = ((temperatue - 32) * 5) / 9;
System.out.println("Temperatue in Celsius is : " + temperatue);
scanner.close();
}
}
Enter temperatue in Fahrenheit :
150
Temperatue in Celsius is : 65.55556
Java Program to swap two numbers using a third variable
package com.asyncster.java.program.examples;
/* Java Program to swap two numbers using a third variable .
*/
import java.util.Scanner;
public class SwapTwoNumbers {
public static void main(String args[]) {
int num1, num2, temp;
System.out.println("Enter value for num1 and num2 : ");
Scanner scanner = new Scanner(System.in);
num1 = scanner.nextInt();
num2 = scanner.nextInt();
System.out.println("Before Swapping num1 is: " + num1 + " and num2 is:" + num2);
temp = num1;
num1 = num2;
num2 = temp;
System.out.println("After Swapping num1 is: " + num1 + "\n and num2 is : " + num2);
scanner.close();
}
}
Enter the value for num1 and num2 :
34 105
Before Swapping num1 is: 34 and num2 is:105
After Swapping num1 is: 105
and num2 is: 34
Java Program to swap two numbers without using a third variable
package com.asyncster.java.program.examples;
/* Java Program to swap two numbers without using third variable
*/
import java.util.Scanner;
public class SwapTwoNumbers2 {
public static void main(String args[]) {
int num1, num2;
System.out.println("Enter value for num1 and num2: ");
Scanner scanner = new Scanner(System.in);
num1 = scanner.nextInt();
num2 = scanner.nextInt();
System.out.println("Before Swapping num1 is : " + num1 + " and num2 is :" + num2);
num1 = num1 + num2;
num2 = num1 - num2;
num1 = num1 - num2;
System.out.println("After Swapping num1 is: " + num1 + " and num2 is : " + num2);
scanner.close();
}
}
Enter value for num1 and num2:
34 78
Before Swapping num1 is : 34 and num2 is :78
After Swapping num1 is: 78 and num2 is : 34
Java Program to find the largest number among three numbers
package com.asyncster.java.program.examples;
/*
Java Program to find the largest number among three numbers
*/
import java.util.Scanner;
public class LargestNumbersAmong3 {
public static void main(String args[]) {
int num1, num2, num3;
System.out.println("Enter three integers ");
Scanner scanner = new Scanner(System.in);
num1 = scanner.nextInt();
num2 = scanner.nextInt();
num3 = scanner.nextInt();
if (num1 > num2 && num1 > num3) {
System.out.println("the first number is largest : "+num1);
} else if (num2 > num1 && num2 > num3) {
System.out.println("the second number is largest: "+num2);
} else if (num3 > num1 && num3 > num2) {
System.out.println("the third number is largest : "+num3);
} else {
System.out.println("entered number are same.");
}
scanner.close();
}
}
Enter three integers
34 89 121
the third number is largest : 121
Java Program to find out the factorial of a given number
package com.asyncster.java.program.examples;
/*
* Java Program to find out the factorial of a given number.
* */
import java.util.Scanner;
public class FactorialOfNumber {
public static void main(String args[]) {
int num, counter, fact = 1;
System.out.println("Enter number to find the Factorial : ");
Scanner scanner = new Scanner(System.in);
num = scanner.nextInt();
if (num < 0) {
System.out.println("Number should be greater than 0.");
} else {
for (counter = 1; counter <= num; counter++) {
fact = fact * counter;
}
System.out.println("Factorial of " + num + " is = " + fact);
}
scanner.close();
}
}
Enter number to find the Factorial :
4
Factorial of 4 is = 24
Java Program to Read Input From User Using Scanner
package com.asyncster.java.program.examples;
/*
Java Program to Read Input From User Using Scanner
* */
import java.util.Scanner;
public class ReadInputFromUsingScanner {
public static void main(String args[]) {
int num;
float salary;
Scanner scanner = new Scanner(System.in);
System.out.println("Enter an integer: ");
num = scanner.nextInt();
System.out.println("You entered integer: " + num);
salary = scanner.nextFloat();
System.out.println("You entered salary as float " + salary);
scanner.close();
}
}
Enter an integer:
12
You entered integer: 12
34.55
You entered salary as float 34.55
Java Program to accept and print Command Line Argument
package com.asyncster.java.program.examples;
/*
Java Program to accept and print Command Line Argument
*/
class CommandLineArguments {
public static void main(String[] args) {
for (String arg : args) {
System.out.println("The arguments are : " + arg);
}
}
}
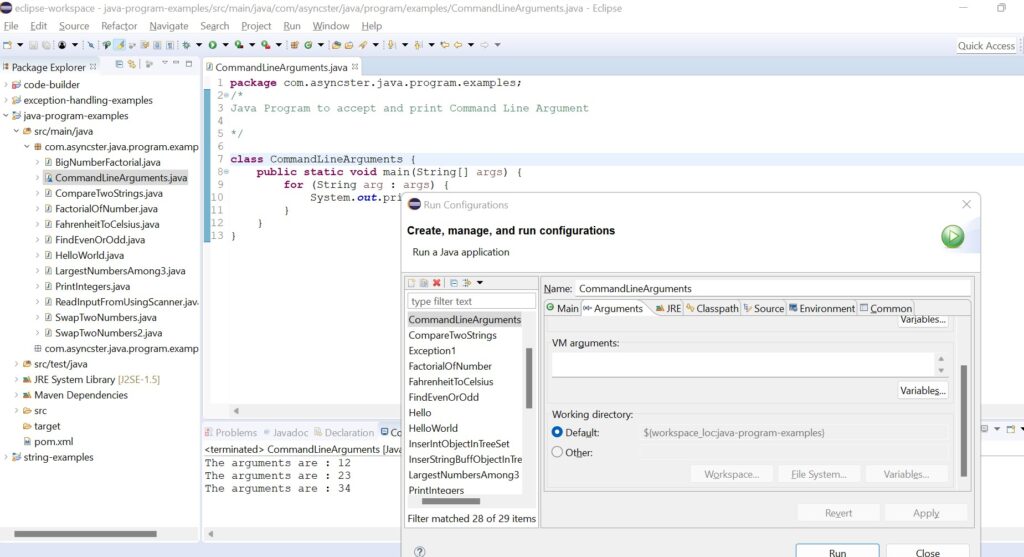
The arguments are : 12
The arguments are : Async
The arguments are : 100.7
The arguments are : false
Java programs to compare two Strings
package com.asyncster.java.program.examples;
/*
Java programs to compare two Strings
*/
import java.util.Scanner;
public class CompareTwoStrings {
public static void main(String args[]) {
String str1, str2;
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the first string : ");
str1 = scanner.nextLine();
System.out.println("Enter the second string : ");
str2 = scanner.nextLine();
if (str1.compareTo(str2) > 0) {
System.out.println("First String is greater :" + str1);
} else if (str1.compareTo(str2) < 0) {
System.out.println("Second string is greater : " + str2);
} else {
System.out.println("Both strings are equal.");
}
scanner.close();
}
}
Enter the first string :
Hello
Enter the second string :
Welcome
Second string is greater : Welcome
Java Program to Calculate factorial for large Numbers using BigIntegers
package com.asyncster.java.program.examples;
/* Java Program to Calculate factorial for large Numbers using BigIntegers */
import java.util.Scanner;
import java.math.BigInteger;
public class BigNumberFactorial {
public static void main(String args[]) {
int num, counter;
BigInteger inc = new BigInteger("1");
BigInteger fact = new BigInteger("1");
Scanner scanner = new Scanner(System.in);
System.out.println("Enter big number to find Factorial : ");
num = scanner.nextInt();
for (counter = 1; counter <= num; counter++) {
fact = fact.multiply(inc);
inc = inc.add(BigInteger.ONE);
}
System.out.println("the Factorial of number "+num+" is: " + fact);
scanner.close();
}
}
Enter big number to find Factorial :
50
the Factorial of number 50 is: 30414093201713378043612608166064768844377641568960512000000000000
Articles related to Java programs interview questions
How we attach Values to Enum in Java
How to check if an enum contains a String
In this article, we have seen basic java programs for building logic and cracking the coding interviews.